Spring Data permette di semplificare lo stato di persistenza rimuovendo completamente l’implementazione dei DAO dalla nostra applicazione. Per fare ciò, l’interfaccia DAO deve estendere JpaRepository e Spring Data creerà automaticamente un’implementazione dotata dei metodi CRUD più rilevanti per l’accesso ai dati.
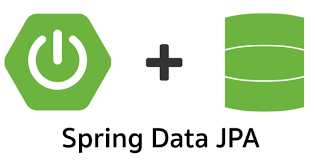
Spring Data Esempio
public interface CountryRepository extends JpaRepository<Country, Integer> { }
Dove Country è l’entity che rappresenta la tabella Country
@Entity @Table(name = "COUNTRY") public class Country { @Id @Column(name = "id") @GeneratedValue(strategy = GenerationType.IDENTITY) int id; @Column(name = "countryName") String countryName; @Column(name = "population") long population; public Country() { super(); } public Country(int i, String countryName, long population) { super(); this.id = i; this.countryName = countryName; this.population = population; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getCountryName() { return countryName; } public void setCountryName(String countryName) { this.countryName = countryName; } public long getPopulation() { return population; } public void setPopulation(long population) { this.population = population; } }
Il controller usa normalmente CountryRepository come un normale dao
@RestController @RequestMapping("/api") public class HelloController { @Autowired private CountryRepository repository; // ------------------- Ricerca Per Nome ------------------------------------ //api/ciao @RequestMapping(value = "/cercaid/{countryId}", method = RequestMethod.GET, produces = "application/json") public ResponseEntity<Optional<Country>> ricercaPerId(@PathVariable("countryId") Integer countryId) { Optional<Country> res = repository.findById(countryId); return new ResponseEntity<Optional<Country>>(res, HttpStatus.OK); } }
Dal codice, si può notare che repository ha un’implementazione di findById creata direttamente da Spring Data.
I metodi forniti di default sono tanti, sul sito ufficiale si trovare una abbondante documentazione:
Spesso si necessità di costruire delle query ad hoc. Anche in questo caso fa tutto Spring Data.
public interface CountryRepository extends JpaRepository<Country, Integer> { List<Country> findByCountryName(String countryName); @Query(value = "SELECT * FROM COUNTRY WHERE COUNTRY_NAME LIKE :cl", nativeQuery = true) List<Country> SelByDescrizioneLike(@Param("cl") String cl); //Query JPQL @Query(value = "FROM Country WHERE countryName LIKE :cl") List<Country> SelByDescrizioneLikeJPQL(@Param("cl") String cl); @Transactional @Modifying @Query(value = "DELETE FROM COUNTRY WHERE COUNTRY_NAME = :cl", nativeQuery = true) void DelRowCountryName(@Param("cl") String cl); }
Serve una ricerca di country per cognome? È sufficiente mettere la firma del metodo, ovviamente con il nome corretto:
List<Country> findByCountryName(String countryName);
Per eseguire una query sql nativa, basta inserire l’annotation @query, con il parametro native settato a true:
@Query(value = "SELECT * FROM COUNTRY WHERE COUNTRY_NAME LIKE :cl", nativeQuery = true) List<Country> SelByDescrizioneLike(@Param("cl") String cl);
altrimenti la query è considerata scritta in JPQL:
//Query JPQL @Query(value = "FROM Country WHERE countryName LIKE :cl") List<Country> SelByDescrizioneLikeJPQL(@Param("cl") String cl);
Nel caso si abbia necessità di modificare il db, va startata una transazione:
@Transactional @Modifying @Query(value = "DELETE FROM COUNTRY WHERE COUNTRY_NAME = :cl", nativeQuery = true) void DelRowCountryName(@Param("cl") String cl);
Download Codice Progetto:
https://programmingacademy.it/wp-content/uploads/2020/11/HelloWorldSpringBootDataJpa.zip